In one of my previous projects, I was responsible for designing a system to manage and track order lifecycles. We needed a robust way to capture every change an order went through—right from creation to delivery. Essentially, every state transition like “Order Created”, “Order Paid”, “Order Packed”, “Order Shipped”, and “Order Delivered” needed to be tracked reliably.
I had two technical choices in mind:
- Use MongoDB with a separate collection to store events.
- Use EventStoreDB, a purpose-built database for event sourcing.
After careful evaluation, I chose EventStoreDB—and here’s why.
Table of Contents
The Temptation: MongoDB with Event Collections
At first glance, MongoDB seemed more than capable. I could use one collection for current order state (orders) and another for tracking events (order_events). Each event document would contain the order ID, event type, timestamp, and metadata.
{
“order_id”: “ORD123”,
“event_type”: “OrderPacked”,
“timestamp”: “2025-05-20T10:00:00Z”,
“actor”: “admin”
}
It looked clean and was easy to query. But the deeper I went, the more I uncovered fundamental challenges with this approach.
The Hidden Costs of Event Tracking in MongoDB
While MongoDB is excellent for flexible data storage and fast reads/writes, using it for event sourcing revealed several problems:
1. Dual-Write Complexity: You have to write to both the current state (orders) and event (order_events) collections. If one write succeeds and the other fails, you lose data consistency. MongoDB does offer transactions, but using them increases latency and adds complexity.
2. Replaying State is Manual and Costly: Want to reconstruct the current state from events? You’ll need to:
- Fetch all events for an order
- Sort them
- Apply business rules to rehydrate state
EventStoreDB does this natively. MongoDB does not.
3. No Native Concurrency Control: If two services try to update the same order simultaneously, race conditions can occur unless you manually handle versioning. EventStoreDB uses optimistic concurrency by default and rejects conflicting writes.
4. Limited Support for Subscriptions & Replay: MongoDB’s change streams are decent, but they don’t offer the same fidelity or features as EventStoreDB’s persistent subscriptions and replay capabilities.
What Makes EventStoreDB Stand Out?
EventStoreDB is a database specifically designed for event sourcing. In this pattern, every change in the system is captured as an immutable event, and current state is derived from those events.
1. Immutable Log of Events: Each order becomes a stream of events, and you can always go back and see exactly how it evolved. No hidden changes, no overwritten data.
2. Built-in Projections: You can define projections (views of data) that get automatically updated as new events are written. These help generate read models for APIs or dashboards.
3. High Performance Replay: If business rules change, you can replay historical events through a new projection to create a new read model—without touching the existing database.
4. Subscriptions and Catch-up Readers: Services can subscribe to streams and react in real time when new events occur. This powers event-driven microservices, automation, and real-time monitoring.
Real-World Use Cases Across Industries
Event sourcing and EventStoreDB aren’t just for niche tech projects. Many companies across industries use them to gain auditability, traceability, and future-proof architecture.
Retail & E-Commerce
- Use Case: Order lifecycle, payments, returns
- How It Helps: Every customer interaction, status change, or refund is tracked as an event. In case of a customer dispute or system failure, you can reconstruct the full order history with timestamps.
Example: A global e-commerce platform uses EventStoreDB to handle 5 million events per day for orders and inventory. Any order can be reconstructed instantly for support or compliance.
Banking & Fintech
- Use Case: Transaction history, ledger management
- How It Helps: Traditional CRUD systems update balances directly. Event sourcing tracks every transaction as an immutable event, creating a verifiable audit trail.
Example: A digital wallet service adopted EventStoreDB to comply with financial regulations by keeping a full event log of every balance change and action.
Logistics & Supply Chain
- Use Case: Shipment tracking, fleet status
- How It Helps: Packages change location, ownership, or condition frequently. By logging each change as an event, companies gain complete traceability from origin to delivery.
Example: A logistics startup uses EventStoreDB to track every parcel’s journey. In case of a lost shipment, they replay the event stream to trace exactly where things went wrong.
Healthcare
- Use Case: Patient record versioning, treatment updates
- How It Helps: Medical records are sensitive. Event sourcing guarantees no past data is lost or tampered with. Treatments, prescriptions, and doctor notes become traceable events.
Example: A hospital management system uses EventStoreDB to ensure every patient change is recorded immutably—helping comply with HIPAA and improving diagnostics.
AI & Analytics
- Use Case: Training data audit, feature evolution
- How It Helps: In ML pipelines, knowing why a model made a prediction often depends on knowing the exact input state. Event sourcing provides the raw truth to reconstruct state at prediction time.
Example: An AI firm uses EventStoreDB to track input events and user behavior changes, helping debug model drift and maintain reproducibility.
Numbers That Matter
Metric | MongoDB with Manual Events | EventStoreDB |
Max Events/sec | ~5,000–10,000 (depending on indexing) | 15,000+ events/sec per node |
Event Replay Latency | High (manual code to replay and hydrate) | Very Low (built-in projections) |
Storage Overhead | High (need for redundant current state) | Lower (single source of truth) |
Recovery Accuracy | Moderate | Perfect replayability |
Developer Time Spent | 3x more to build/maintain event logic | Lower—features come built-in |
Final Verdict: EventStoreDB Is Worth It
While MongoDB can technically handle event storage, the overhead of maintaining consistency, replay logic, and concurrency control makes it a poor long-term fit for systems where auditing, accuracy, and traceability are critical.
EventStoreDB gives you a powerful foundation for modern architectures:
- Audit trails by default
- Event-driven integrations
- Easier debugging and forensics
- Smooth scalability with eventual consistency
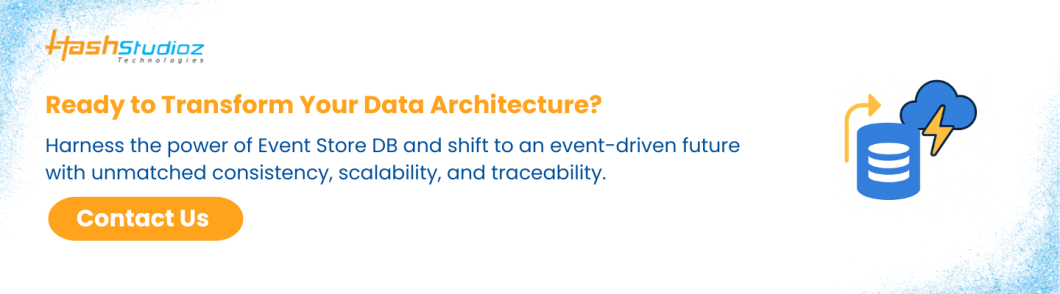
Conclusion
If you’re designing a system where the journey matters as much as the destination—order processing, financial transactions, logistics, etc.—EventStoreDB offers you the tools and guarantees MongoDB doesn’t.
By choosing EventStoreDB, we future-proofed our architecture, empowered downstream systems with reliable events, and gave stakeholders a complete, trusted view of order histories.